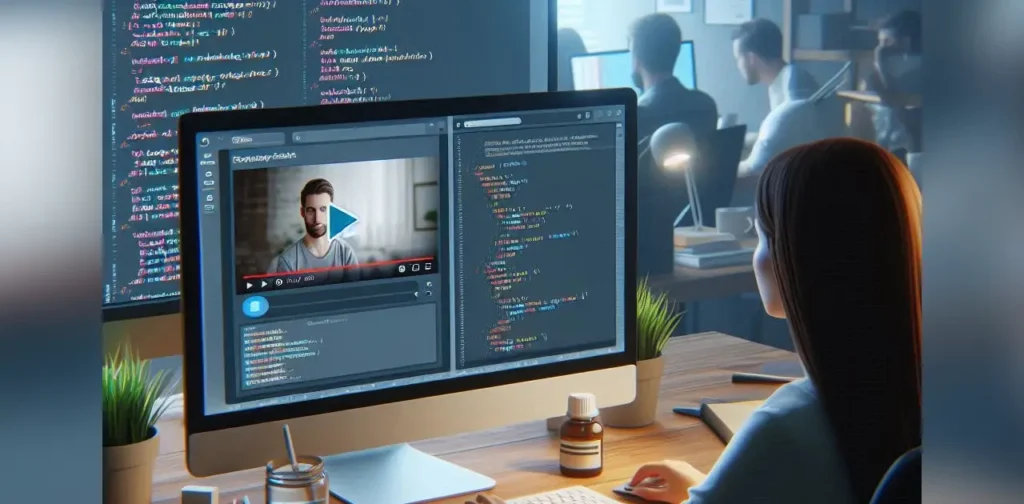
Play Pause Video on Scroll with JavaScript through a webpage can greatly enhance user engagement and interactivity. This effect, achieved using JavaScript, is not only visually appealing but also simple to implement. In this guide, I’ll walk you through the process step by step, and I’ll provide free code that you can use on your website.
What You’ll Learn in This Article
- Auto Play Pause Video on Scroll with JavaScript.
- Implementing the effect using JavaScript with an easy-to-follow example.
- How the code works explained in plain language.
Free Code to Play Pause Video on Scroll with JavaScript
Here’s the code you’ll need to get started. This example ensures the video pauses when the page is scrolled and updates the video frame to match the scroll position. Copy and paste it into your HTML file to see it in action.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Play Pause Video on Scroll</title>
<style>
#page_height {
display: block;
height: 10500px;
}
#video_area {
position: fixed;
top: 0;
left: 0;
width: 100%;
}
p {
font-size: 24px;
font-family: Arial, Helvetica, sans-serif;
}
</style>
</head>
<body>
<div id="page_height"></div>
<p id="time"></p>
<video id="video_area" tabindex="0" autobuffer="autobuffer" preload="preload">
<source src="your-video-file.mp4" type="video/mp4">
<p>Sorry, your browser does not support the video element.</p>
</video>
<script>
// Select the video element
var vid = document.getElementById('video_area');
// Pause the video initially
vid.pause();
// Pause the video when the user scrolls
window.onscroll = function () {
vid.pause();
};
// Update video frame based on scroll position
setInterval(function () {
vid.currentTime = window.pageYOffset / 200;
}, 80);
</script>
</body>
</html>
Step-by-Step Implementation
1. HTML Structure
The HTML file contains a video element and a dummy div to create scrollable space. Replace your-video-file.mp4 with your actual video file path.
2. CSS Styling
The CSS ensures the video remains fixed at the top of the page. This layout creates an immersive scrolling experience.
3. JavaScript Video Scroll Interaction
Let’s break down the JavaScript code:
- Pause the Video on Load: The line vid.pause(); ensures the video doesn’t start playing automatically.
- Pause on Scroll: The window.onscroll function pauses the video every time the user scrolls.
- Update Frames Dynamically: The setInterval function updates the video frame based on the page’s scroll position. The calculation window.pageYOffset / 200 adjusts the currentTime of the video.
Why This Code is Useful
This code is lightweight and doesn’t require external libraries. It ensures a smooth, user-friendly experience. Whether you’re building a portfolio or creating an interactive webpage, this feature can add a professional touch.
FAQs for Play Pause Video on Scroll with JavaScript
1. Can I use this code for other video formats?
Yes, you can! Replace the source element’s type with the appropriate MIME type for your video format (e.g., video/mp4, video/ogg).
2. What happens if the video is too long?
Adjust the calculation in window.pageYOffset / 200 to sync the video length with your page’s scroll height.
3. Is this compatible with all browsers?
This code works with modern browsers like Chrome, Firefox, and Edge. For older browsers, test compatibility or include a fallback message.
Table: JavaScript Functions Used
Function/Method | Purpose | Example |
---|---|---|
document.getElementById | Selects an HTML element by its ID. | var vid = document.getElementById('video_area'); |
vid.pause() | Pauses the video. | vid.pause(); |
window.onscroll | Triggers an event when the user scrolls. | window.onscroll = function () {...} |
setInterval | Repeats a function at a set interval. | setInterval(function() {...}, 80); |
Pro Tip: Enhance User Experience
For better performance:
- Compress videos to reduce loading time.
- Use a video player library for advanced controls.
By implementing this tutorial, you’ll be able to add an interactive and professional feature to your website with minimal effort. Give it a try and watch your site’s interactivity soar!