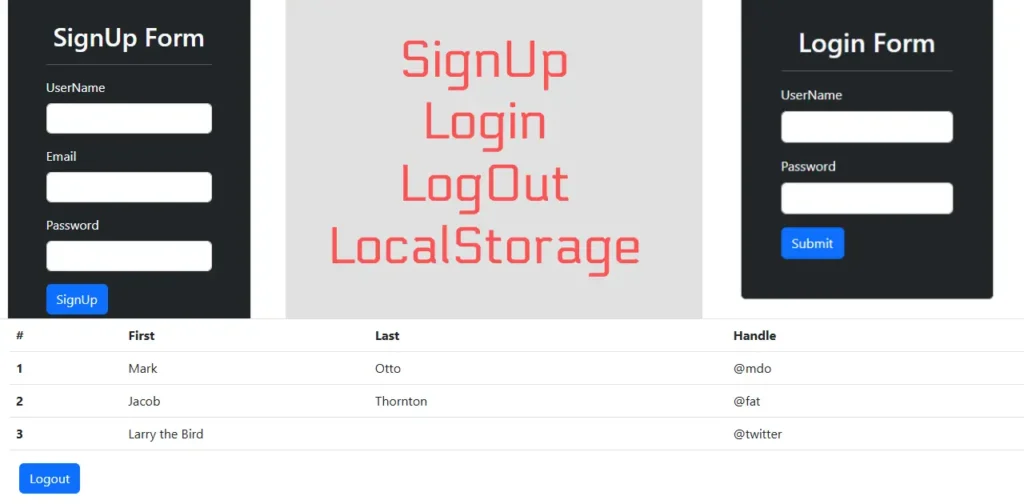
Creating a JavaScript Login Form with Signup and Logout is an essential project to learn how to handle user authentication using localStorage. This article walks you through the process step by step, explains the code, and provides a free downloadable package containing all the files. Let’s get started!
What You’ll Learn
- Signup Form: To collect user details and store them securely using localStorage.
- Login Form: To validate user credentials and authenticate the user.
- Logout Functionality: To manage sessions and log out users.
- Access Control: Ensure only authenticated users can view certain data.
Free Code Package
You can download the complete code package here to explore and implement this project. It contains four files:
- index.html: Signup page.
- login.html: Login page.
- logout.html: Page for authenticated users with logout functionality.
- script.js: The JavaScript file managing all functionality.
Step-by-Step Guide
1. Signup Form (index.html)
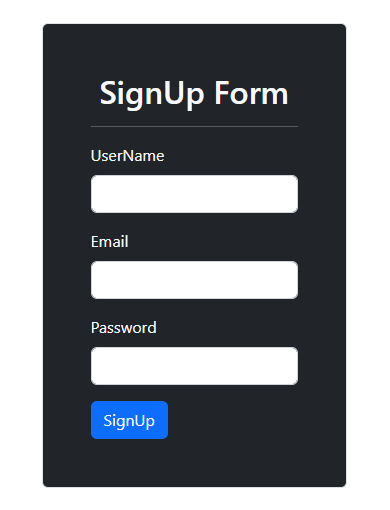
The signup form collects username, email, and password and stores them in localStorage. If any field is empty, it will display an error.
Key Features:
- Validates input fields.
- Redirects users to the login page after successful signup.
Code Explanation:
The following code creates a signup form:
<!doctype html>
<html lang="en">
<head>
<title>SignUp</title>
<!-- Required meta tags -->
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<!-- Bootstrap CSS v5.2.1 -->
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet"
integrity="sha384-iYQeCzEYFbKjA/T2uDLTpkwGzCiq6soy8tYaI1GyVh/UjpbCx/TYkiZhlZB6+fzT" crossorigin="anonymous">
</head>
<body>
<main>
<div class="container">
<div class="row">
<div class="d-flex align-items-center justify-content-center mt-5">
<form class="w-lg-50 border p-5 bg-dark text-light rounded" method="post">
<h2 class="text-center">SignUp Form</h2>
<hr>
<div class="mb-3">
<label for="username" class="form-label">UserName</label>
<input type="text" class="form-control" id="username" name="username" required>
</div>
<div class="mb-3">
<label for="email" class="form-label">Email</label>
<input type="email" class="form-control" id="email" name="email" required>
</div>
<div class="mb-3">
<label for="password" class="form-label">Password</label>
<input type="password" class="form-control" id="password" name="password" required>
</div>
<button type="submit" class="btn btn-primary" id="submit" onclick="signup(event)">SignUp</button>
</form>
</div>
</div>
</div>
</main>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.min.js"
integrity="sha384-7VPbUDkoPSGFnVtYi0QogXtr74QeVeeIs99Qfg5YCF+TidwNdjvaKZX19NZ/e6oz" crossorigin="anonymous">
</script>
<script src="script.js"></script>
</body>
</html>
2. Login Form (login.html)
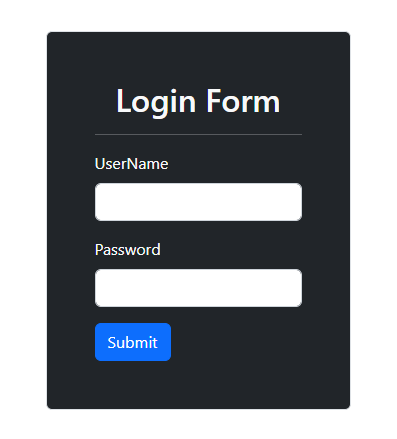
The login form validates the user’s credentials against the data stored in localStorage during signup. If the credentials match, the user is redirected to the logout page.
Key Features:
- Displays alerts for incorrect credentials.
- Stores a token in localStorage to manage the session.
Code Explanation:
Here’s how the login form works:
<!doctype html>
<html lang="en">
<head>
<title>Login</title>
<!-- Required meta tags -->
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<!-- Bootstrap CSS v5.2.1 -->
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet"
integrity="sha384-iYQeCzEYFbKjA/T2uDLTpkwGzCiq6soy8tYaI1GyVh/UjpbCx/TYkiZhlZB6+fzT" crossorigin="anonymous">
</head>
<body>
<main>
<div class="container">
<div class="row">
<div class="d-flex align-items-center justify-content-center mt-5">
<form class="w-lg-50 border p-5 bg-dark text-light rounded" method="post">
<h2 class="text-center">Login Form</h2>
<hr>
<div class="mb-3">
<label for="username" class="form-label">UserName</label>
<input type="text" class="form-control" id="loginUsername" name="username" required>
</div>
<div class="mb-3">
<label for="password" class="form-label">Password</label>
<input type="password" class="form-control" id="loginPassword" name="password" required>
</div>
<button type="submit" class="btn btn-primary" id="submit" onclick="login(event)">Submit</button>
</form>
</div>
</div>
</div>
</main>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.min.js"
integrity="sha384-7VPbUDkoPSGFnVtYi0QogXtr74QeVeeIs99Qfg5YCF+TidwNdjvaKZX19NZ/e6oz" crossorigin="anonymous">
</script>
<script src="script.js"></script>
</body>
</html>
3. Logout Page (logout.html)

The logout page is accessible only to authenticated users. It displays a table with some dummy data and includes a logout button that clears the session.
Key Features:
- Displays secret data after login.
- Provides a logout button to end the session.
Code Explanation:
Here’s the table and logout button:
<!doctype html>
<html lang="en">
<head>
<title>Logout</title>
<!-- Required meta tags -->
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no">
<!-- Bootstrap CSS v5.2.1 -->
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet"
integrity="sha384-iYQeCzEYFbKjA/T2uDLTpkwGzCiq6soy8tYaI1GyVh/UjpbCx/TYkiZhlZB6+fzT" crossorigin="anonymous">
</head>
<body>
<div class="container">
<div class="row">
<table class="table">
<thead>
<tr>
<th scope="col">#</th>
<th scope="col">First</th>
<th scope="col">Last</th>
<th scope="col">Handle</th>
</tr>
</thead>
<tbody>
<tr>
<th scope="row">1</th>
<td>Mark</td>
<td>Otto</td>
<td>@mdo</td>
</tr>
<tr>
<th scope="row">2</th>
<td>Jacob</td>
<td>Thornton</td>
<td>@fat</td>
</tr>
<tr>
<th scope="row">3</th>
<td colspan="2">Larry the Bird</td>
<td>@twitter</td>
</tr>
</tbody>
</table>
</div>
<button class="btn btn-primary" onclick="logOut()">Logout</button>
</div>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.min.js"
integrity="sha384-7VPbUDkoPSGFnVtYi0QogXtr74QeVeeIs99Qfg5YCF+TidwNdjvaKZX19NZ/e6oz" crossorigin="anonymous">
</script>
<script>
var localToken = localStorage.getItem('token')
if (localToken=='secret') {
}
else{
window.location.href='login.html'
}
function logOut() {
localStorage.setItem('token', 'logout');
window.location.href='login.html'
}
</script>
</body>
</html>
JavaScript Code (script.js) | JavaScript Login Form with Signup and Logout
This file handles all the logic for signup & login.
Signup Function:
javascript
console.log('attached')
// signUp Form
function signup(event) {
event.preventDefault();
var username = document.getElementById('username').value;
var email = document.getElementById('email').value;
var password = document.getElementById('password').value;
if (username, email, password == '') {
alert('Please fill credentials')
return false
}
else {
localStorage.setItem('usernameSignUp', username)
localStorage.setItem('emailSignUp', email)
localStorage.setItem('passwordSignUp', password)
username = document.getElementById('username').value = '';
email = document.getElementById('email').value = '';
password = document.getElementById('password').value = '';
alert('User register successfully!!!')
window.location.href = 'login.html'
}
}
// login form
function login(event) {
event.preventDefault();
var loginEmail = document.getElementById('loginUsername').value;
var loginPassword = document.getElementById('loginPassword').value;
var email1 = localStorage.getItem('usernameSignUp')
var pwd1 = localStorage.getItem('passwordSignUp')
if (email1 == loginEmail && pwd1 == loginPassword) {
alert('done')
localStorage.setItem('token', 'secret');
window.location.href = 'logout.html'
}
else {
alert('Username and password is incorrect')
}
}
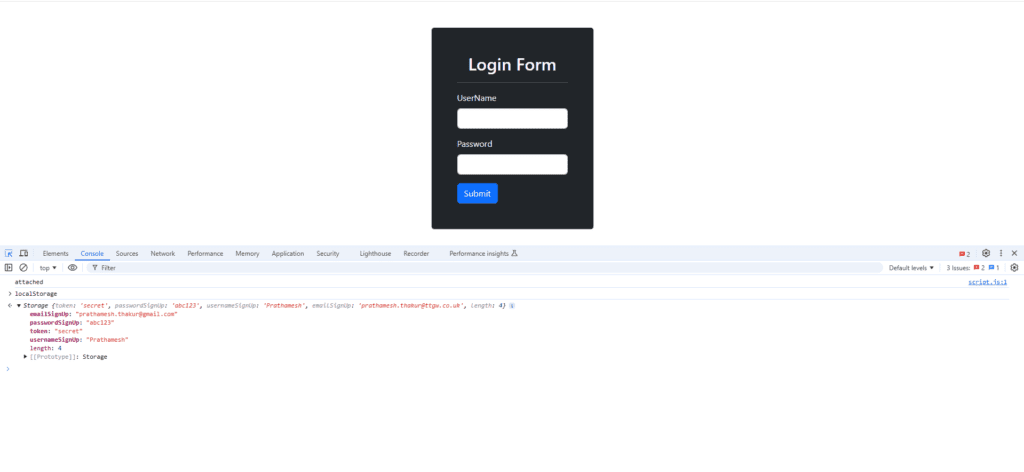
Table of Features
Feature | Description |
---|---|
Signup Form | Collects user details and stores them in localStorage. |
Login Form | Authenticates the user and redirects to the logout page. |
Logout Functionality | Clears the session and redirects back to the login page. |
LocalStorage Usage | Securely stores user data and session tokens. |
FAQs for JavaScript Login Form with Signup and Logout
Q1. What is localStorage?
LocalStorage is a web storage feature that allows you to store data in the user’s browser without expiration.
Q2. Can I use this code for real applications?
This project is for learning purposes. For real-world applications, use secure server-side storage and authentication.
Q3. What happens if I clear the browser’s localStorage?
If localStorage is cleared, all stored user data will be lost, and you will need to sign up again.
Here you can download whole code and run it your browser. Just click on Download button you will receive zip file.
Conclusion
Building a JavaScript Login Form with Signup and Logout is an excellent way to practice web development skills. Download the complete code package here and start your project today. Customize it as per your requirements and add additional security features for a more robust application.